Using Redis for Inter-Process Communication in Robotics, IoT, and AI Applications: A Quick Guide with Python Examples
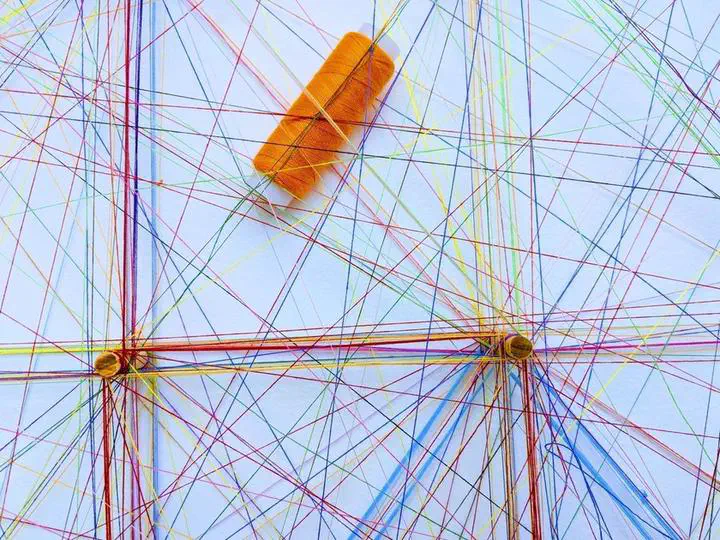
Redis, an in-memory data structure store, can be an effective tool for inter-process communication (IPC) in robotics, IoT, and AI applications. This guide will show you how to use Redis with Python to share data and facilitate communication in different applications.
We can quickly start a Redis instance with Docker:
docker run -p 6379:6379 -it redis/redis-stack:latest
Use pip
to install Redis Python package:
pip install redis
In robotics, Redis can share data between processes that control the robot’s movement, perception, and autonomy. For example, sensor data can be stored in a Redis cache and updated in real-time, allowing the robot’s behaviour process to access the most recent data. Here’s an example of how to store sensor data in a Redis cache using Python:
import redis
# Connect to the Redis server
r = redis.Redis()
# Store sensor data in the Redis cache
r.set("Temperature", 25)
r.set("Humidity", 0.5)
Additionally, Redis can facilitate communication between multiple robots, allowing them to share information and coordinate their actions. Here’s an example of how to use Redis’s pub/sub feature to send a message from one robot to another:
import redis
# Connect to the Redis server
r = redis.Redis()
# Send a message to the "robot_communication" channel
r.publish("robot_communication", "Robot 1 moving to position X")
In IoT, Redis can share data between different devices and applications. For example, sensor data from IoT devices can be stored in a Redis cache and accessed by various applications for real-time monitoring and analysis. Here’s an example of how to retrieve sensor data from a Redis cache using Python:
import redis
# Connect to the Redis server
r = redis.Redis()
# Retrieve sensor data from the Redis cache
temperature = r.get("Temperature")
humidity = r.get("Humidity")
print(temperature, humidity) # Output: 25, 0.5
In AI applications, Redis can be used to share data between different processes that are used for training and inference. For example, the training process can store the model parameters in a Redis cache, and the inference process can access the most recent parameters. Here’s an example of how to store model parameters in a Redis cache using Python:
import redis
# Connect to the Redis server
r = redis.Redis()
# Store model parameters in the Redis cache
r.set("learning_rate", 0.01)
r.set("batch_size", 32)
Overall, Redis offers a fast, flexible, and reliable solution for IPC in robotics, IoT, and AI applications. Its in-memory data storage allows for low-latency access to real-time data, making it an ideal choice for high-performance and real-time processing applications. With Redis and Python, you can easily share and process data between different parts of your system, whether between robots, IoT devices, or AI models, making it possible to create more advanced and innovative systems.